11. ProgressBar¶
The Gtk.ProgressBar
is typically used to display the progress of a
long running operation. It provides a visual clue that processing is underway.
The Gtk.ProgressBar
can be used in two different modes: percentage
mode and activity mode.
When an application can determine how much work needs to take place (e.g. read
a fixed number of bytes from a file) and can monitor its progress, it can use
the Gtk.ProgressBar
in percentage mode and the user sees a growing
bar indicating the percentage of the work that has been completed.
In this mode, the application is required to call Gtk.ProgressBar.set_fraction()
periodically to update the progress bar, passing a float between 0 and 1 to provide
the new percentage value.
When an application has no accurate way of knowing the amount of work to do, it
can use activity mode, which shows activity by a block moving back and forth
within the progress area. In this mode, the application is required to call
Gtk.ProgressBar.pulse()
periodically to update the progress bar.
You can also choose the step size, with the Gtk.ProgressBar.set_pulse_step()
method.
By default, Gtk.ProgressBar
is horizontal and left-to-right, but you
can change it to a vertical progress bar by using the
Gtk.ProgressBar.set_orientation()
method. Changing the direction the
progress bar grows can be done using Gtk.ProgressBar.set_inverted()
.
Gtk.ProgressBar
can also contain text which can be set by calling
Gtk.ProgressBar.set_text()
and Gtk.ProgressBar.set_show_text()
.
11.1. Example¶
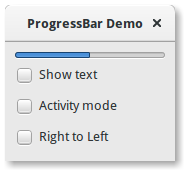
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 | import gi gi.require_version("Gtk", "3.0") from gi.repository import Gtk, GLib class ProgressBarWindow(Gtk.Window): def __init__(self): super().__init__(title="ProgressBar Demo") self.set_border_width(10) vbox = Gtk.Box(orientation=Gtk.Orientation.VERTICAL, spacing=6) self.add(vbox) self.progressbar = Gtk.ProgressBar() vbox.pack_start(self.progressbar, True, True, 0) button = Gtk.CheckButton(label="Show text") button.connect("toggled", self.on_show_text_toggled) vbox.pack_start(button, True, True, 0) button = Gtk.CheckButton(label="Activity mode") button.connect("toggled", self.on_activity_mode_toggled) vbox.pack_start(button, True, True, 0) button = Gtk.CheckButton(label="Right to Left") button.connect("toggled", self.on_right_to_left_toggled) vbox.pack_start(button, True, True, 0) self.timeout_id = GLib.timeout_add(50, self.on_timeout, None) self.activity_mode = False def on_show_text_toggled(self, button): show_text = button.get_active() if show_text: text = "some text" else: text = None self.progressbar.set_text(text) self.progressbar.set_show_text(show_text) def on_activity_mode_toggled(self, button): self.activity_mode = button.get_active() if self.activity_mode: self.progressbar.pulse() else: self.progressbar.set_fraction(0.0) def on_right_to_left_toggled(self, button): value = button.get_active() self.progressbar.set_inverted(value) def on_timeout(self, user_data): """ Update value on the progress bar """ if self.activity_mode: self.progressbar.pulse() else: new_value = self.progressbar.get_fraction() + 0.01 if new_value > 1: new_value = 0 self.progressbar.set_fraction(new_value) # As this is a timeout function, return True so that it # continues to get called return True win = ProgressBarWindow() win.connect("destroy", Gtk.main_quit) win.show_all() Gtk.main() |